この記事のねらい
GA4 page_view イベントのパラメータ、 page_referrer のデータを探索からCSVダウンロードして、ChatGPT に Pythonのコードを書いてもらって、視覚化したいと思います。
なお今回は、ChatGPT に Pythonのコードを書いてもらうだけで、ChatGPT APIは使用しておりません。また、BigQueryは使用せず、GA4の探索からCSVダウンロードした、page_referrerとpage_locationのデータを使用する想定です。
既にご存じの方も多いと思いますが、ChatGPTは自然言語で「こういうコードを作りたい」と話しかけると、様々なプログラミング言語をたいてい数十秒で書いてくれます。
今回はPythonです。また、もしも実環境にてコードを実行してエラーが出たら、エラーを貼り付けることで、修正箇所の指摘を行ってくるだけでなく、全体を一から書き直すこともやってくれます。今回は、ChatGPT PLUS(月額約20ドル)を使用しました。
なお、GA4とPythonについてのご質問は、弊社GA4 無料コミュニティ(リンク⇒●)までお願いいたします。Discordなので、ハンドルネームで気軽にご参加ください。
ステップ1: シンプルなサンプルデータ
まずは、シンプルなサンプルデータとして、以下のようなテーブルデータをCSVで用意しました。
1列目は、今回の主役、page_referrer=ひとつ前に見たURL(の一部であるパス)です。
2列目は、現在見ているページのパスです。
3列目は、1列目から2列目へと進んだ閲覧数です。
今回は、Pythonのプログラミングをすることが目的なので、下記のサンプルデータで、まずは実行させていただきます。
Previous_URL | Current_URL | Transition_Count |
---|---|---|
/aaa.html | /ccc.html | 524 |
/aaa.html | /ddd.html | 34 |
/aaa.html | /eee.html | 685 |
/bbb.html | /aaa.html | 686 |
/bbb.html | /ccc.html | 167 |
/bbb.html | /ddd.html | 896 |
/bbb.html | /eee.html | 241 |
/ccc.html | /aaa.html | 660 |
/ccc.html | /bbb.html | 379 |
/ccc.html | /ddd.html | 589 |
/ccc.html | /eee.html | 279 |
/ddd.html | /aaa.html | 104 |
/ddd.html | /bbb.html | 718 |
/ddd.html | /ccc.html | 665 |
/ddd.html | /eee.html | 78 |
/eee.html | /aaa.html | 841 |
/eee.html | /bbb.html | 835 |
/eee.html | /ccc.html | 370 |
/eee.html | /ddd.html | 263 |
上記のデータ貼り付けて、ChatGPT PLUS に以下の質問をしてみました。
なお、ChatGPTに質問する時は、実データではなく、サンプルデータを作成して質問することをオススメします。
GA4からダウンロードできる、以下のCSVデータを使って、ウェブサイト内のユーザーの動きを可視化したい。
# CSVデータの与件
- GA4からダウンロードできる
- カラム1: ユーザーが一つ前に見たページのURL
- カラム2: ユーザーが現在見ているページのURL
- カラム3: カラム1のURLからカラム2のURLに遷移した遷移数
# プログラムの与件
- Pythonを使用して処理する
- ユーザーの動きを可視化する
- 処理前のデータは c:\PythonSampleFiles\Download_from_GA4.csv
- 処理後のデータは c:\PythonSampleFiles\Visualized_by_Python.csv
- テーブルの列名は、Previous_URL, Current_URL, Transition_Count
上記の与件で、ChatGPTにコードを書いてもらいました。簡潔なコメント文も入れてくれます。
import pandas as pd
import networkx as nx
import matplotlib.pyplot as plt
# CSVファイルを読み込む
data = pd.read_csv('c:\\PythonSampleFiles\\Download_from_GA4.csv')
# 列名を変更する
data = data.rename(columns={'Previous_URL': 'Previous URL', 'Current_URL': 'Current URL', 'Transition_Count': 'Weight'})
# ページ間の遷移数を集計する
transitions = data.groupby(['Previous URL', 'Current URL']).size().reset_index(name='Weight')
# ページをノードとしたグラフを作成する
G = nx.DiGraph()
for i, row in transitions.iterrows():
G.add_edge(row['Previous URL'], row['Current URL'], weight=row['Weight'])
# ノードのサイズを設定する
sizes = {node: transitions['Weight'][transitions['Previous URL'] == node].sum() for node in G.nodes()}
sizes = [sizes[node] * 10 for node in G.nodes()]
# グラフを可視化する
pos = nx.spring_layout(G)
nx.draw(G, pos, node_size=sizes, with_labels=True, edge_color='gray', arrows=True, arrowstyle='->', width=1, font_size=10)
# グラフを保存する
plt.savefig('c:\\PythonSampleFiles\\Visualized_by_Python.png')
このコードでは、まずCSVファイルを読み込んで、前のページと現在のページのペアごとに遷移数を集計しています。次に、ページをノードとした有向グラフを作成し、ノードのサイズを遷移数の合計に応じて調整しています。最後に、matplotlibとnetworkxを使用してグラフを描画し、可視化した結果をPNGファイルとして保存しています。
なお、このコードを実行するには、matplotlibとnetworkxというライブラリをインストールする必要があります。以下のコマンドを使用してインストールしてください。
pip install matplotlib networkx
読者の皆様が実際にお試しになる場合は、コード内の下記の部分を書き換えてください。
# CSVファイルを読み込む
data = pd.read_csv('c:\\PythonSampleFiles\\Download_from_GA4.csv')
# グラフを保存する
plt.savefig('c:\\PythonSampleFiles\\Visualized_by_Python.png')
実際に図を描いて見ると、下記のような図を描くことができました。しかしこれでは、地味過ぎて、遷移数も分かません。
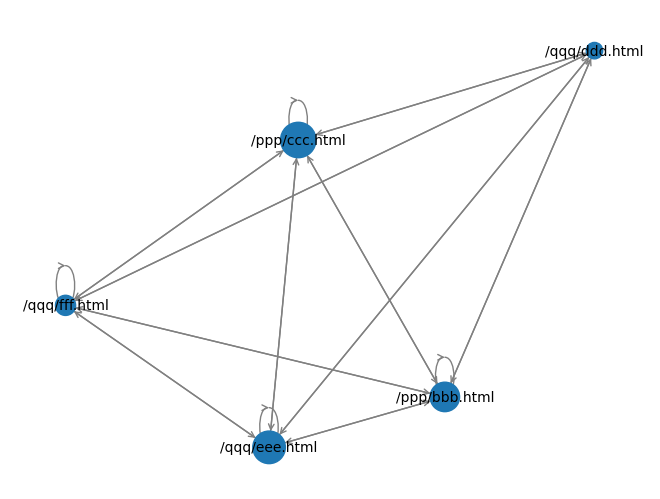
ChatGPTには、これまでの流れを踏まえて、追加で質問や依頼をすることができます。Slackで人間と会話するような感じです。
以下の仕様の追加をお願いします。
・色分けしてカラフルに見せる
・線で表現されている部分にそれぞれに該当する値の数値を入れる
・該当する値によって、線の太さを変える(実際にやって見ると、線が太過ぎたので、下記コードでは、デフォルトで描画される太さの100分の1になるように調整しています。)
ということで、上記の依頼をして、ChatGPT PLUSにコードを書いてもらいました。
import pandas as pd
import networkx as nx
import matplotlib.pyplot as plt
# CSVファイルを読み込む
data = pd.read_csv('c:\\PythonSampleFiles\\Download_from_GA4.csv')
# 列名を変更する
data = data.rename(columns={'Previous_URL': 'Previous URL', 'Current_URL': 'Current URL', 'Transition_Count': 'Weight'})
# ページ間の遷移数を集計する
transitions = data.groupby(['Previous URL', 'Current URL']).sum('Weight').reset_index()
# ページをノードとしたグラフを作成する
G = nx.DiGraph()
for i, row in transitions.iterrows():
G.add_edge(row['Previous URL'], row['Current URL'], weight=row['Weight'])
# ノードのサイズを設定する
sizes = {node: data['Weight'][data['Previous URL'] == node].sum() for node in G.nodes()}
sizes = [sizes[node] for node in G.nodes()]
# 色のリストを作成する
edge_colors = [float(w) for w in transitions['Weight']]
# エッジの太さを設定する
edge_widths = [float(w) for w in transitions['Weight']]
edge_widths = [w * 0.01 for w in edge_widths]
# グラフを可視化する
pos = nx.spring_layout(G)
nx.draw(G, pos, node_size=sizes, with_labels=True, edge_color=edge_colors, cmap=plt.cm.Blues, arrows=True, arrowstyle='->', width=edge_widths, font_size=10)
# エッジの重みに応じて数値を表示する
labels = {}
for i, row in transitions.iterrows():
key = (row['Previous URL'], row['Current URL'])
labels[key] = str(row['Weight'])
nx.draw_networkx_edge_labels(G, pos, edge_labels=labels, font_size=8)
# カラーバーを追加する
sm = plt.cm.ScalarMappable(cmap=plt.cm.Blues, norm=plt.Normalize(vmin=min(edge_colors), vmax=max(edge_colors)))
sm.set_array([])
cbar = plt.colorbar(sm)
cbar.ax.set_ylabel('Edge weight')
# グラフを保存する
plt.savefig('c:\\PythonSampleFiles\\Visualized_by_Python.png')
生成された図は、こちらです。最初に生成された図より、分かりやすくなりましたね。
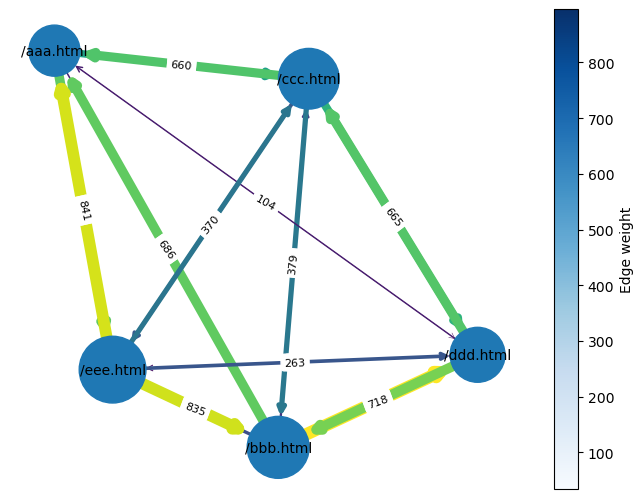
今回は、サンプルデータを使った作図でしたので、次回は実データで挑戦したいと思います。
冒頭にも書かせて頂きましたが、GA4とPythonについてのご質問は、弊社GA4 無料コミュニティまでお願いいたします。Discordなので、ハンドルネームで気軽にご参加ください。
コメント